第一步打开示例代码并参考论坛链接。连接mqtt
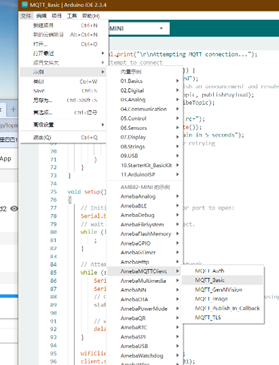
【新提醒】【安信可小安派BW21-CBV-Kit + 数据上云服务器 MQTT方式】 - 小安派·BW21-CBV-KIt - 物联网开发者社区-安信可论坛 - Powered by Discuz!
https://bbs.ai-thinker.com/forum.php?mod=viewthread&tid=46005

第二步修改代码
/*
MQTT Basic example
This sketch demonstrates the basic capabilities of the library.
- connects to an MQTT server
- publishes "hello world" to the topic "outTopic"
- subscribes to the topic "inTopic", printing out any messages it receives. It assumes the received payloads are strings not binary
It will reconnect to the server if the connection is lost using a blocking
reconnect function. See the 'mqtt_reconnect_nonblocking' example for how to
achieve the same result without blocking the main loop.
Example guide:
https://www.amebaiot.com/en/amebapro2-arduino-mqtt-upload-listen/
*/
#include <WiFi.h>
#include <PubSubClient.h>
char ssid[] = "你的WiFi名称"; // your network SSID (name)
char pass[] = "你的WiFi密码"; // your network password
int status = WL_IDLE_STATUS; // Indicator of Wifi status
char mqttServer[] = "bemfa.com";
char clientId[] = "你的私钥";
char publishTopic[] = "XIAOAN2";
char publishPayload[] = "hello world";
char subscribeTopic[] = "XIAOAN3";
// 模拟传感器数据
float temperature = 25.0; // 初始温度
float humidity = 60.0; // 初始湿度
void callback(char* topic, byte* payload, unsigned int length)
{
Serial.print("Message arrived [");
Serial.print(topic);
Serial.print("] ");
for (unsigned int i = 0; i < length; i++) {
Serial.print((char)(payload[i]));
}
Serial.println();
}
WiFiClient wifiClient;
PubSubClient client(wifiClient);
void reconnect()
{
// Loop until we're reconnected
while (!(client.connected())) {
Serial.print("\r\nAttempting MQTT connection...");
// Attempt to connect
if (client.connect(clientId)) {
Serial.println("connected");
// Once connected, publish an announcement and resubscribe
client.publish(publishTopic, publishPayload);
client.subscribe(subscribeTopic);
} else {
Serial.println("failed, rc=");
Serial.print(client.state());
Serial.println(" try again in 5 seconds");
// Wait 5 seconds before retrying
delay(5000);
}
}
}
void setup()
{
// Initialize serial and wait for port to open:
Serial.begin(115200);
// wait for serial port to connect.
while (!Serial) {
;
}
// Attempt to connect to WiFi network
while (status != WL_CONNECTED) {
Serial.print("\r\nAttempting to connect to SSID: ");
Serial.println(ssid);
// Connect to WPA/WPA2 network. Change this line if using open or WEP network:
status = WiFi.begin(ssid, pass);
// wait 10 seconds for connection:
delay(10000);
}
wifiClient.setNonBlockingMode();
client.setServer(mqttServer, 9501);
client.setCallback(callback);
// Allow Hardware to sort itself out
delay(1500);
}
void loop()
{
if (!client.connected()) {
reconnect();
}
client.loop();
// 模拟传感器数据更新
temperature += random(-1, 2); // 随机变化温度
humidity += random(-2, 3); // 随机变化湿度
// 创建JSON格式的数据
char payload[128];
snprintf(payload, sizeof(payload), "{\"temperature\": %.1f, \"humidity\": %.1f}", temperature, humidity);
// 发布数据到MQTT服务器
client.publish(publishTopic, payload);
// 每5秒发送一次数据
delay(5000);
}
第三步配置fuxa
第1步。硬件。
需要一个树莓派。并且安装好系统。
树莓派系统镜像版本
2024-03-12-raspios-bullseye-arm64.img.xz
树莓派系统镜像烧录工具
imager_1.8.5.exe
第2步安装docker
参考https://pidoc.cn/docs/pidoc/install_docker/
卸载旧版本(如果有):
for pkg in docker.io docker-doc docker-compose podman-docker containerd runc; do sudo apt-get remove $pkg; done
添加 Docker 官方 GPG key:
sudo apt-get update
sudo apt-get install ca-certificates curl
sudo install -m 0755 -d /etc/apt/keyrings
sudo curl -fsSL http://mirrors.tencent.com/docker-ce/linux/debian/gpg -o /etc/apt/keyrings/docker_tencentyun.asc
sudo chmod a+r /etc/apt/keyrings/docker_tencentyun.asc
添加仓库到 Apt 源:
echo
"deb [arch=$(dpkg --print-architecture) signed-by=/etc/apt/keyrings/docker_tencentyun.asc] http://mirrors.tencent.com/docker-ce/linux/debian
(. /etc/os-release && echo "VERSION_CODENAME") stable" |
sudo tee /etc/apt/sources.list.d/docker_tencentyun.list > /dev/null
sudo apt-get update
国内加速镜像 pull 失败问题
创建或修改 /etc/docker/daemon.json 文件,并重启 docker 服务。
sudo mkdir -p /etc/docker
sudo tee /etc/docker/daemon.json <<-'EOF'
{
"registry-mirrors": ["https://docker.pidoc.tech"]
}
EOF
sudo systemctl daemon-reload
sudo systemctl restart docker
- 安装 Docker 软件包
要安装最新版本,请运行:
sudo apt-get install docker-ce docker-ce-cli containerd.io docker-buildx-plugin docker-compose-plugin
- 验证
通过运行镜像验证安装是否成功hello-world
sudo docker run hello-world
此命令下载测试映像并在容器中运行它。当容器运行时,它会打印一条确认消息并退出。
第3步配置docker图形化工具。
配置docker图形化工具portainer
拉取镜像
sudo docker pull portainer/portainer
创建 portainer 容器
sudo docker volume create portainer_data
运行 portainer
sudo docker run -d -p 9000:9000 --name portainer --restart always -v /var/run/docker.sock:/var/run/docker.sock -v portainer_data:/data portainer/portainer
运行之后在浏览器中输入树莓派IP:9000 进入界面。首次访问需要设定登录密码。
第4步安装fuxa
FUXA——基于Web的过程可视化软件
sudo docker pull frangoteam/fuxa:latest
启动一个后台运行的容器,将容器内部的 1881 端口映射到宿主机的 1881 端口
// persistent storage of application data (project), daq (tags history) and logs
docker run -d -p 1881:1881 -v fuxa_appdata:/usr/src/app/FUXA/server/_appdata -v fuxa_db:/usr/src/app/FUXA/server/_db -v fuxa_logs:/usr/src/app/FUXA/server/_logs frangoteam/fuxa:latest
第5步设置fuxa自动启动。
当容器启动时fuxa也会自动启动,保证断电重新启动时fuxa会自动启动。
sudo docker run -d -p 1881:1881 --restart=always frangoteam/fuxa:latest
第三步配置fuxa连接mqtt
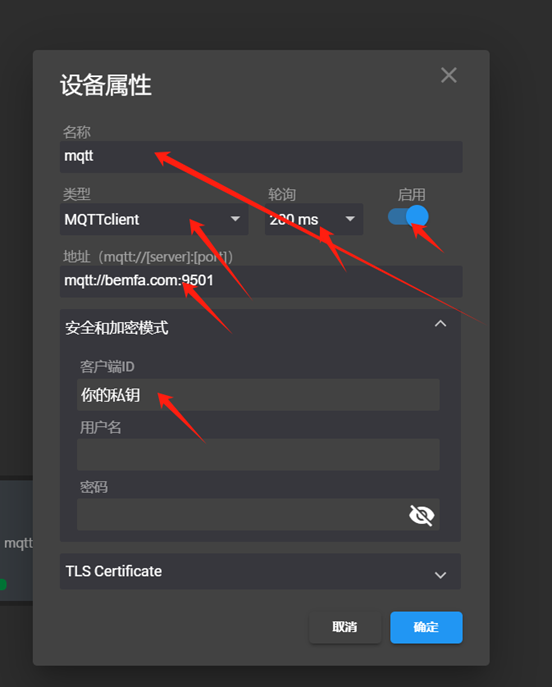
连接成功标志
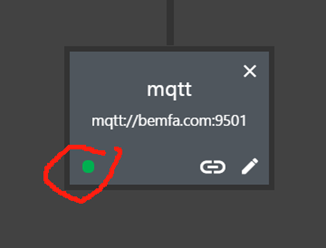
添加变量链接
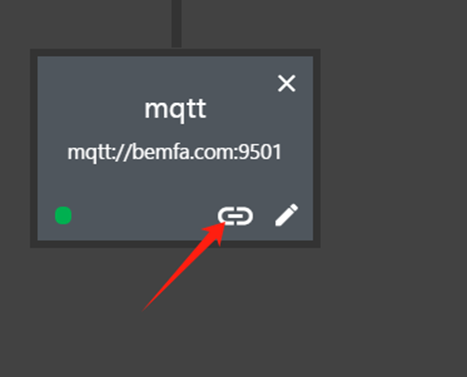
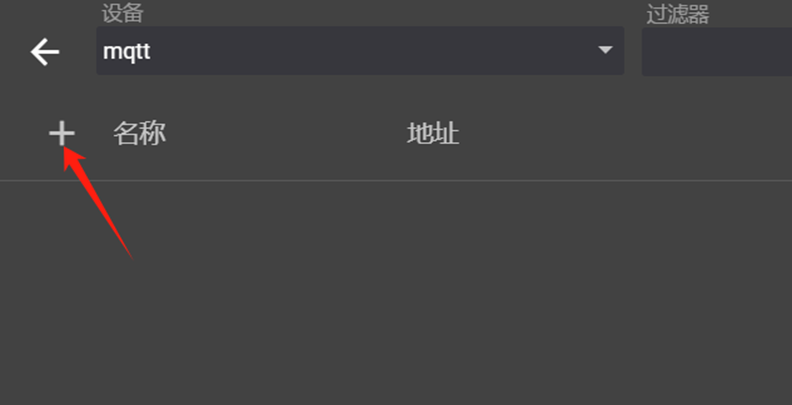
添加主题
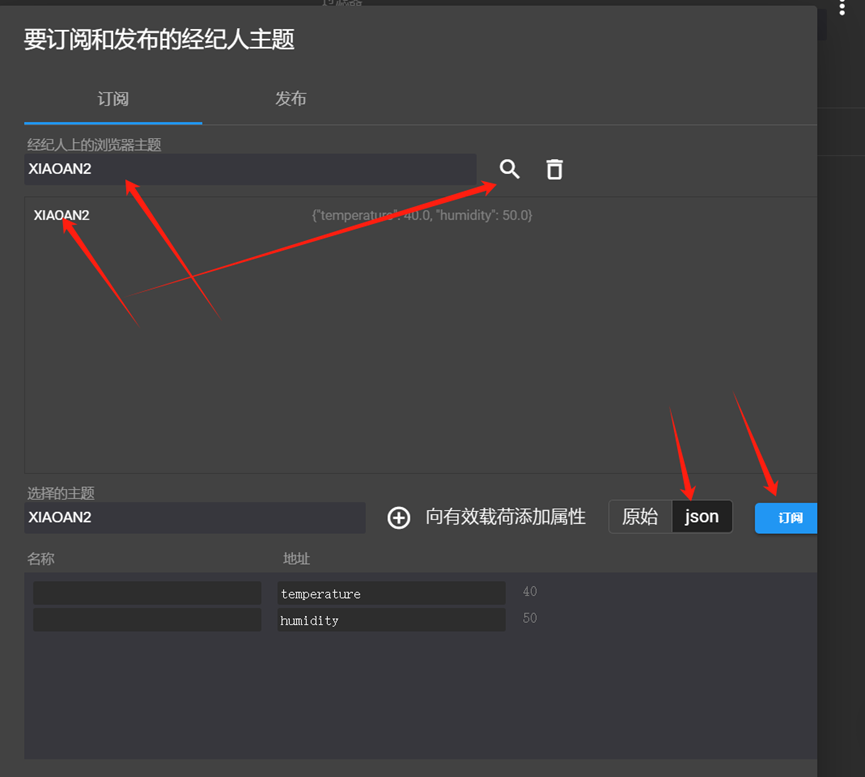
已经采集到变量值

绘制图表
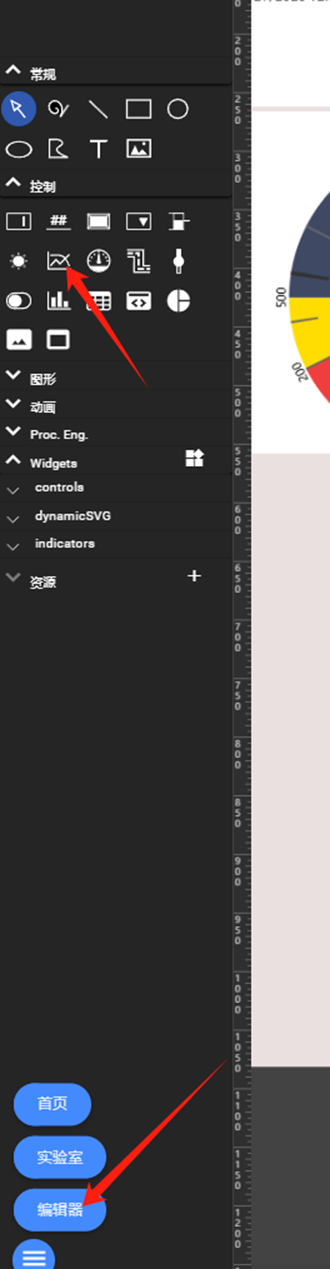
图表内数据关联变量
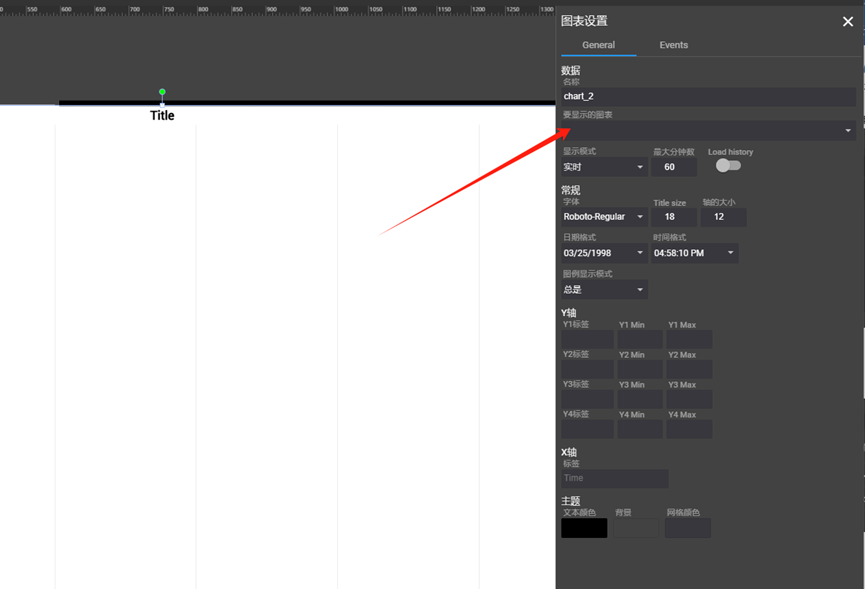
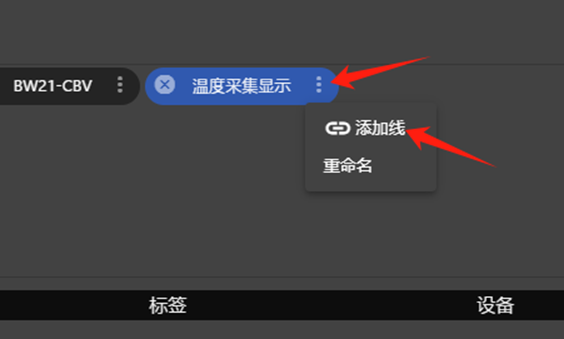
给表格起一个名字
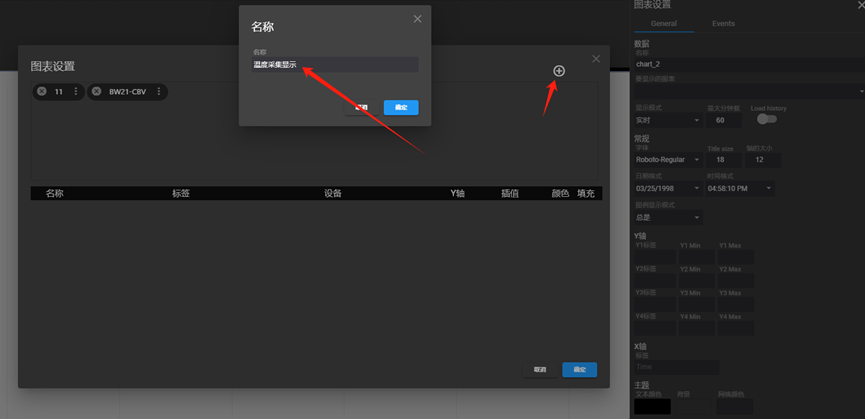
添加曲线的变量标签
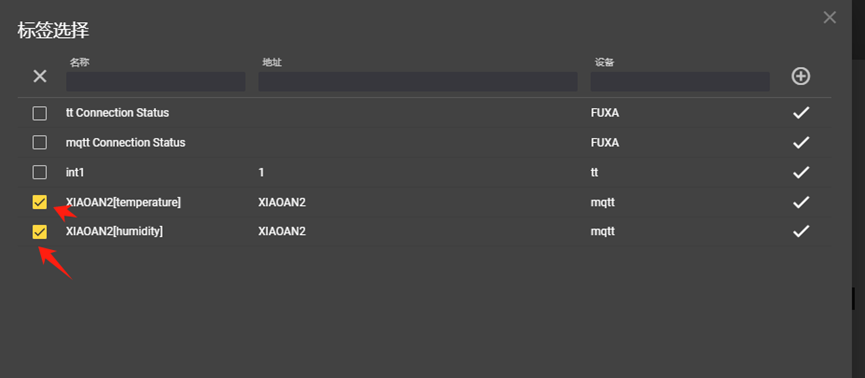
点击运行键
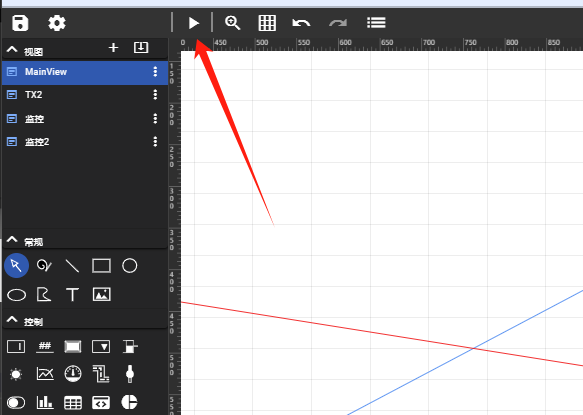
你就会得到模拟曲线
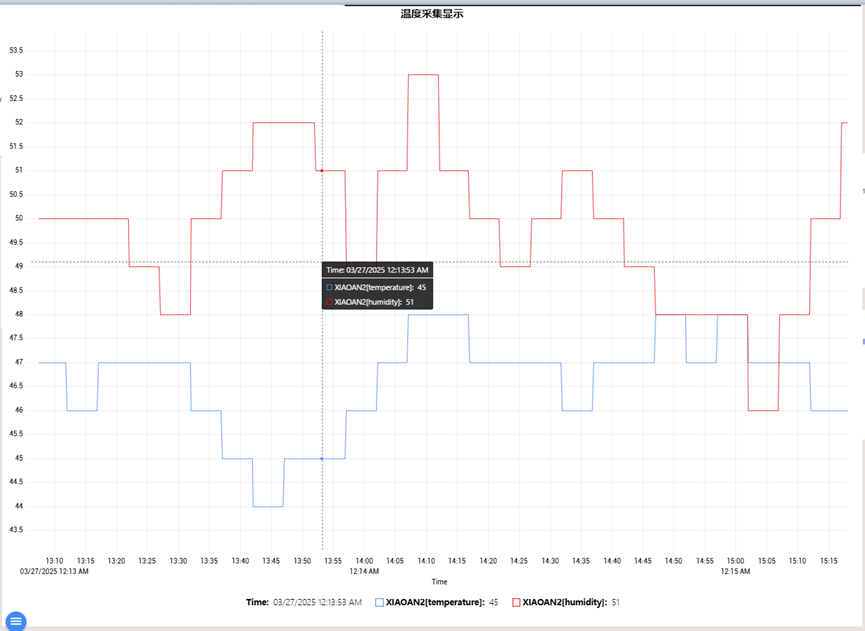
将来看是否可以将视频嵌入到fuxa,那样就更有价值。











